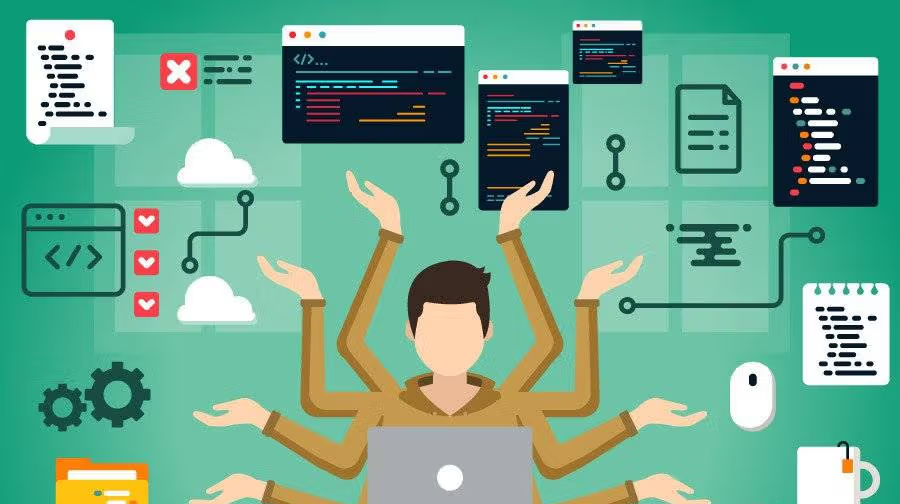
Understanding Asynchronous Programming in JavaScript
Asynchronous programming can seem complex, but it’s essential for building efficient web applications. If you’re taking full stack developer classes, understanding how asynchronous programming works in JavaScript is crucial. It allows you to handle tasks that take time to complete, such as fetching data from a server, without freezing your application. Let’s break down the key concepts and practices to get you up to speed.
What is Asynchronous Programming?
Asynchronous programming enables your code to perform tasks in the background while continuing to execute other code. This is especially useful when dealing with operations that take time, like network requests or reading files. Instead of waiting for these tasks to complete, your application can continue to run smoothly.
Callback Functions
- Basics of Callbacks
Callbacks are functions passed as arguments to other functions. They are executed after the main function is completed. For example, you might use a callback to handle the result of an API request once it finishes. This allows you to manage tasks that depend on the completion of other operations.
- Callback Hell
While callbacks are useful, they can lead to “callback hell” if overused. This happens when you nest multiple callbacks, making the code hard to read and maintain. It’s essential to manage callbacks properly to avoid this issue.
Promises
- Introduction to Promises
Promises represent the eventual completion (or failure) of a typical asynchronous operation and its resulting value. They provide a cleaner way to handle asynchronous tasks compared to nested callbacks. A promise can be in 1 of 3 states: pending, fulfilled, or rejected.
- Using Promises
Promises are used to chain asynchronous operations together. You can use .then() to handle the result of a promise. catch() to handle errors. This chaining makes your code more readable and easier to manage.
Async/Await
- Simplifying Asynchronous Code
Async/await is a syntax introduced in ES2017 that simplifies working with promises. It enables you to generate asynchronous code that appears synchronous, making it easier to read and maintain. Using async before a function makes it return a promise, and await pauses the function until the promise is resolved.
- Error Handling with Async/Await
Error handling is straightforward with async/await. You can utilize try and catch blocks to handle errors, similar to synchronous code. This makes managing exceptions in asynchronous operations much easier.
Event Loop and Concurrency
- How Does an Event Loop Work?
The event loop is a highly critical component of JavaScript’s concurrency model. It allows JavaScript to perform non-blocking operations by pushing tasks to a queue and executing them one at a time. Understanding the event loop helps in managing asynchronous tasks efficiently
- Microtasks and Macrotasks
The event loop handles microtasks and microtasks differently. Microtasks include promise callbacks and are executed before the next macrotask. Macrotasks include tasks like I/O operations and timers. Knowing this distinction helps in optimizing your code and managing execution order.
Practical Applications
- Fetching Data from APIs
One common use of asynchronous programming is fetching data from APIs. You can use fetch or libraries like Axios to make requests. Handling these requests asynchronously ensures your application remains responsive while waiting for data.
- Real-Time Updates
Asynchronous programming is also useful for real-time updates, such as chat applications or live feeds. WebSockets and other real-time technologies can benefit from async/await and promise to manage continuous data streams.
Best Practices
- Avoiding Callback Hell
To avoid callback hell, use promises or async/await. Refactor deeply nested callbacks into separate functions or use async functions to flatten the code structure.
- Error Handling
Always handle errors in your asynchronous code. Whether using promises or async/await, ensure you include proper error handling to manage failures gracefully and provide a better user experience.
Performance Considerations
- Minimizing Blocking Operations
Avoid blocking operations that can delay your application. Use asynchronous functions for tasks like file reading or network requests to keep your application responsive.
- Optimizing Event Loop Performance
Be mindful of how tasks are queued and executed. Optimizing your code to minimize the time spent in the event loop can improve your application’s performance and responsiveness.
Conclusion
Understanding asynchronous programming is vital for any full stack developer. Whether you’re enrolled in a Java full stack developer course, mastering these concepts will enhance your ability to build efficient and responsive web applications. From callbacks and promises to async/await, each approach has its place in modern JavaScript development. By applying these techniques and various best practices, you can create applications that handle complex asynchronous tasks with ease.
Business Name: ExcelR – Full Stack Developer And Business Analyst Course in Bangalore
Address: 10, 3rd floor, Safeway Plaza, 27th Main Rd, Old Madiwala, Jay Bheema Nagar, 1st Stage, BTM 1st Stage, Bengaluru, Karnataka 560068
Phone: 7353006061
Business Email: enquiry@excelr.com